Java 中 抽象类 和 接口的不同点
众所周知,抽象是指隐藏功能的内部实现,只向用户显示功能。 例如:只显示它是做什么的,隐藏其如何实现的。 抽象类on)和接口都用于抽象,因此接口和抽象类是抽象的必需先决条件
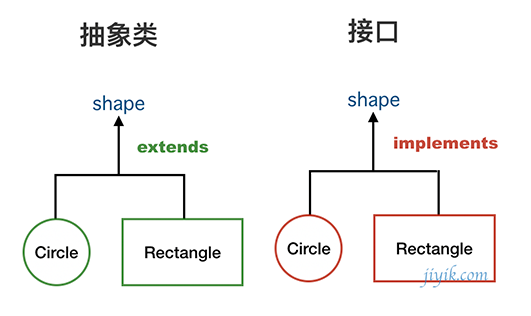
- 方法类型 :接口只能有抽象方法。抽象类可以有抽象方法和非抽象方法。从 Java 8 开始,它也可以有默认和静态方法。
- 最终变量 :Java 接口中声明的变量默认为最终变量。抽象类可能包含非最终变量。
- 变量类型 :抽象类可以有 final、非final、静态和非静态变量。接口只有静态和 final 变量。
- 实现 :抽象类可以提供接口的实现。接口不能提供抽象类的实现。
- 继承与抽象 :Java 接口可以使用关键字“implements”来实现,抽象类可以使用关键字“extends”来继承。
- 多个实现 :一个接口只能继承另一个Java接口,一个抽象类可以继承另一个Java类并实现多个Java接口。
- 数据成员的可访问性 :默认情况下,Java 接口的成员是 public 的。 Java 抽象类可以具有 private、protected 等类成员。
下面我们来看一个示例
import java.io.*;
abstract class Shape {
String objectName = " ";
Shape(String name) { this.objectName = name; }
public void moveTo(int x, int y)
{
System.out.println(this.objectName + " "
+ "has been moved to"
+ " x = " + x + " and y = " + y);
}
abstract public double area();
abstract public void draw();
}
class Rectangle extends Shape {
int length, width;
Rectangle(int length, int width, String name)
{
super(name);
this.length = length;
this.width = width;
}
@Override public void draw()
{
System.out.println("Rectangle has been drawn ");
}
@Override public double area()
{
return (double)(length * width);
}
}
class Circle extends Shape {
double pi = 3.14;
int radius;
Circle(int radius, String name)
{
super(name);
this.radius = radius;
}
@Override public void draw()
{
System.out.println("Circle has been drawn ");
}
@Override public double area()
{
return (double)((pi * radius * radius));
}
}
public class GFG {
public static void main(String[] args)
{
Shape rect = new Rectangle(2, 3, "Rectangle");
System.out.println("Area of rectangle: "
+ rect.area());
rect.moveTo(1, 2);
System.out.println(" ");
Shape circle = new Circle(2, "Circle");
System.out.println("Area of circle: "
+ circle.area());
circle.moveTo(2, 4);
}
}
上面的示例输出结果如下
Area of rectangle: 6.0
Rectangle has been moved to x = 1 and y = 2
Area of circle: 12.56
Circle has been moved to x = 2 and y = 4
如果我们在矩形和圆形之间没有任何共同的代码怎么办,可以使用接口。
import java.io.*;
interface Shape {
void draw();
double area();
}
class Rectangle implements Shape {
int length, width;
Rectangle(int length, int width)
{
this.length = length;
this.width = width;
}
@Override public void draw()
{
System.out.println("Rectangle has been drawn ");
}
@Override public double area()
{
return (double)(length * width);
}
}
class Circle implements Shape {
double pi = 3.14;
int radius;
Circle(int radius) { this.radius = radius; }
@Override public void draw()
{
System.out.println("Circle has been drawn ");
}
@Override public double area()
{
return (double)((pi * radius * radius));
}
}
public class GFG {
public static void main(String[] args)
{
Shape rect = new Rectangle(2, 3);
System.out.println("Area of rectangle: "
+ rect.area());
Shape circle = new Circle(2);
System.out.println("Area of circle: "
+ circle.area());
}
}
上面示例编译执行结果如下
Area of rectangle: 6.0
Area of circle: 12.56
什么时候用接口,什么时候用抽象类?
如果下面中的任何一个适用于你的情况,考虑使用抽象类:
- 在java应用程序中,有一些相关的类需要共享一些代码,那么可以将这些代码行放在抽象类中,这个抽象类应该由所有这些相关类继承。
- 可以在抽象类中定义非静态或非final字段,以便通过方法访问和修改它们所属对象的状态。
- 可以在继承抽象类的类中设置许多 public 方法或字段,或者需要公共以外的访问修饰符(例如受保护和私有)。
如果下面中的任何一个适用于你的情况,考虑使用接口:
- 它是完全抽象的,接口中声明的所有方法都必须由实现该接口的类来实现。
- 一个类可以实现多个接口。它被称为多重继承。
- 想指定特定数据类型的行为,但不关心谁实现了它的行为。
相关文章
在 Java 中获取文件大小
发布时间:2023/05/01 浏览次数:139 分类:Java
-
Java 提供了不同的方法来获取文件的字节大小。 本教程演示了在 Java 中获取文件大小的不同方法。使用 Java IO 的文件类获取文件大小 Java IO 包的 File 类提供了以字节为单位获取文件大小的功能。
Java 中的文件分隔符
发布时间:2023/05/01 浏览次数:108 分类:Java
-
本篇文章介绍了 Java 中的文件分隔符。Java 中的文件分隔符 文件分隔符是用来分隔目录的字符; 例如,Unix 使用 /,Windows 使用 \ 作为文件分隔符。
Java 中的文件过滤器
发布时间:2023/05/01 浏览次数:193 分类:Java
-
本篇文章介绍如何在 Java 中使用 FileFilter。FileFilter 用于过滤具有特定扩展名的文件。 Java内置包IO和Apache Commons IO为FileFilter提供了类和接口来进行文件过滤操作。
Java 获取 ISO 8601 格式的当前时间戳
发布时间:2023/05/01 浏览次数:132 分类:Java
-
本篇文章介绍了 ISO 8601 日期格式、其重要性及其在 Java 中的使用。 它还列出了一些优点来强调为什么应该使用 ISO 格式来表示日期。
在 Java 中获取数组的子集
发布时间:2023/05/01 浏览次数:142 分类:Java
-
本篇文章介绍了几种在 Java 中获取数组子集的方法。使用 Arrays.copyOf() 方法获取数组的子集 使用 Arrays.copyOfRange() 方法获取数组的子集
用 Java 填充二维数组
发布时间:2023/05/01 浏览次数:110 分类:Java
-
二维数组是基于表结构的,即行和列,填充二维数组不能通过简单的添加到数组操作来完成。 本篇文章介绍如何在 Java 中填充二维数组。
计算 Java 数组中的重复元素
发布时间:2023/05/01 浏览次数:202 分类:Java
-
本篇文章介绍Java计算数组中重复元素的方法。计算 Java 数组中的重复元素。我们可以创建一个程序来计算数组中的重复元素。 该数组可以是未排序的,也可以是已排序的。
Java 中 List 和 Arraylist 的区别
发布时间:2023/05/01 浏览次数:90 分类:Java
-
表示为单个单元的一组单个对象称为集合。 在 Java 中,Collection 是一个具有多个已定义接口和类的框架,用于将一组对象表示为一个单元。 它允许我们操纵